Value noise is a type of procedural noise that is commonly used in computer graphics, especially in texture generation, terrain modeling, and various visual effects. The main idea behind value noise is simple but powerful: generate a grid of random values (often between -1 and 1) at integer points, then interpolate between these values to achieve smooth transitions across the grid.
The result is a smooth, continuous field that looks somewhat similar to clouds or waves, depending on the interpolation technique used. Value noise is not as structured or organic as Perlin noise but is much simpler to compute and can be modified easily.
How Value Noise Works
- Random Grid Generation: Set random values at each integer point in a grid. These points serve as the core values from which the noise will be interpolated.
- Interpolation: For any point in between the grid points, interpolate between the nearest grid values. Common interpolation techniques include linear, cosine, or cubic interpolation, which helps create smooth transitions.
- Fractal Summing (Optional): Often, we layer several instances of value noise at different frequencies and amplitudes to create more complex, detailed textures. This layering is known as fractal or octaved noise.
Applications of Value Noise
- Terrain Generation: It’s widely used in generating landscapes for games or simulations. By modifying frequency and amplitude, we can control terrain roughness, creating anything from gentle hills to rugged mountains.
- Water and Cloud Effects: Value noise can simulate cloud cover, ocean waves, and other natural, semi-random phenomena.
- Texture Generation: Due to its organic look, value noise is commonly used for procedural textures (e.g., marble, stone).
- Animated Effects: Its continuous, smooth nature allows value noise to be animated seamlessly, producing flowing visuals like smoke or plasma.
Value Noise vs. Perlin Noise
Although value noise and Perlin noise produce somewhat similar visual results, they are quite different in their core concepts and outputs.
Feature | Value Noise | Perlin Noise |
---|---|---|
Smoothness | Smoother with simpler shapes | More structured with visible patterns |
Algorithm | Uses a grid of random values and interpolates | Uses gradient vectors at grid points for interpolation |
Performance | Generally faster | Slightly slower due to gradient computation |
Use Cases | Basic terrain, textures, and general-purpose noise | More organic effects, terrain, and clouds |
Octaves | Requires more octaves for natural look | Fewer octaves needed for rich detail |
Why Choose One Over the Other?
- Use Value Noise if you need a faster, simpler noise pattern for textures or terrain that doesn’t need highly organic details.
- Use Perlin Noise if you’re looking for natural, flowing patterns, like clouds, fire, or wood grains, that require smooth continuity and directionality.
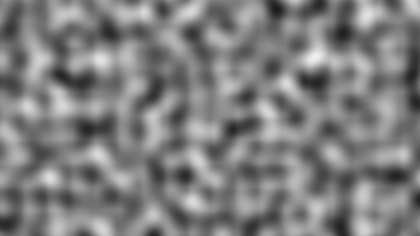
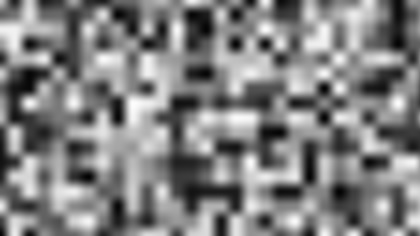
Value noise GLSL implementation in ShaderToy
float random(vec2 st) {
return fract(sin(dot(st.xy, vec2(12.9898, 78.233))) * 43758.5453123);
}
// Smooth interpolation between values using mix
float valueNoise(vec2 uv) {
vec2 i = floor(uv); // Integer grid coordinates
vec2 f = fract(uv); // Fractional part
// Random values at the four corners of the cell
float a = random(i);
float b = random(i + vec2(1.0, 0.0));
float c = random(i + vec2(0.0, 1.0));
float d = random(i + vec2(1.0, 1.0));
// Smooth interpolation for x and y using Hermite curve (smoothstep)
vec2 u = f * f * (3.0 - 2.0 * f);
// Interpolate values along the x and y axis
return mix(mix(a, b, u.x), mix(c, d, u.x), u.y);
}
void mainImage(out vec4 fragColor, in vec2 fragCoord) {
vec2 uv = fragCoord.xy / iResolution.y;
uv *= 16.0; // Scale to increase the frequency of the noise
// Function to hash the integer grid coordinates to random values
// Generate fractal noise with multiple octaves
float noise = 0.0;
float scale = 1.0;
float amplitude = .50;
for (int i = 0; i < 5; i++) { // 5 octaves
noise += valueNoise(uv * scale) * amplitude;
scale *= 2.0;
amplitude *= 0.5;
}
// Set color based on the noise
fragColor = vec4(vec3(noise), 1.0);
}
More Reading
Read about procedural noise functions.
Thanks for reading <3