Coming soon. It is currently preview, whole tutorial will be updated soon.
This is a beginners’ tutorial on making a simple infinite side-scrolling shooter game with basic AI-agents, player mechanics, coins system & high-score system. Part 2 will possibly teach about the bullet shooting system and death system.
I highly recommend the very basic Making your first 2D game tutorial by official Godot engine documentation. That will teach you about the very fundamentals so you will be familiar with the Godot’s UI interface. After that, you will be able to understand this tutorial. As it requires you to be already familiar with the Godot’s interface.
What are we creating?
We will create:
- An airplane that can only move up or down.
- Enemy airplanes that spawn outside the screen and move left towards the player.
- Enemy planes’ speed is unpredictable and variable so it adds extra challange.
- Coins will also spawn outside the screen and move left.
- When player touches a coin, it increments its
coins_count
. - High score will be saved in a file.
- We can re-start the game after we hit the enemy & die.
Setting up the Project
Download Godot Engine. Open it, and create a new project named “Airplane Game”. Once the project created, you should see this:

Creating a Player
We will create our player as a separate scene so the player can be re-used. For this, create a new scene. Right click on the res://
in the file-system (image below) and create a new scene. Name it “Player”.
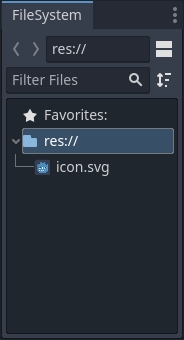
Creating Airplane Sprite
TODO: Work in progress.